What are operators in Java?
Operators are special symbols that are used for performing different kinds of operations. For example, if we want to add two numbers we use the + operator, and if we want to subtract two numbers we use the – operator.
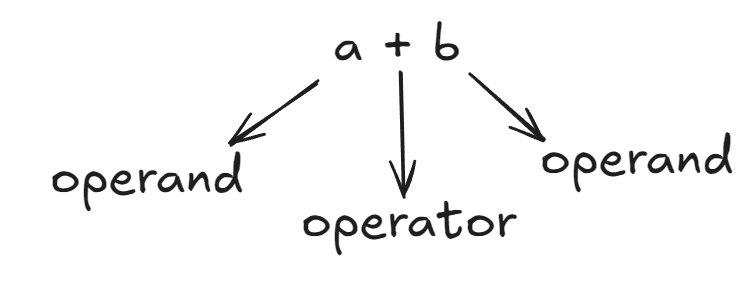
Types of operators in Java:
- Arithmetic operators
- Relational operators
- Logical operators
- Assignment operators
- Unary operators
- Ternary operator
- Bitwise operator
Arithmetic operators
Arithmetic operators are used for performing Arithmetic operations. There are a total of 5 types of Arithmetic operators.
- + Addition
- – Subtraction
- * Multiplication
- / Division
- % Modulus
+ Addition | Addition is used for adding numbers |
– Subtraction | Subtraction is used for Subtracting numbers |
* Multiplication | Subtraction is used for multiplying numbers |
/ Division | Division is used for dividing numbers |
% Modulus | Modulus is used for getting the remainder after the division of numbers |
public class DevExpertsHub {
public static void main(String[] args) {
int a = 20;
int b = 10;
int addition = a + b;
int subtraction = a - b;
int multiplication = a * b;
float division = a / b;
int modulus = a % b;
System.out.println(addition); //output : 30
System.out.println(subtraction); //output : 10
System.out.println(multiplication); //output : 200
System.out.println(division); //output : 2.0
System.out.println(modulus); //output : 0
}
}
This is a code sample for using all Arithmetic operators in Java. I have taken float data type for division because when we divide any two numbers we might get decimal points as a result.
Relational Operators:
Relational operators are used for comparing the operands to determine whether it is equal, greater, or lesser or not equal. There are a total of 6 Relational operators in Java. The result of the outcome will be always either true or false.
== (Equal) | The equal operator is used to check whether the operands are equal If they are equal then it will return true else it will return false. |
!= (Not Equal) | The Not equal operator is used to check whether the operands are not equal If they are not equal then it will return true else it will return false. |
> (Greater than) | The greater than operator is used to check whether the first operand is greater than the second operand If it is greater then it will return true else it will return false. |
< (Smaller than) | The smaller than operator is used to check whether the first operand is smaller than the second operand If it is smaller then it will return true else it will return false. |
>= (Greater than or Equal) | The greater than or equal operator is used to check whether the first operand is greater than or equal to the second operand If it is greater or equal then it will return true else it will return false. |
<= (Smaller than or Equal) | The smaller than or equal operator is used to check whether the first operand is smaller than or equal to the second operand If it is smaller or equal then it will return true else it will return false. |
public class DexExpertsHub {
public static void main(String[] args) {
int a = 20;
int b = 10;
System.out.println(a==b); //output : false
System.out.println(a!=b); //output : true
System.out.println(a>b); //output : true
System.out.println(a<b); //output : false
System.out.println(a>=b); //output : true
System.out.println(a<=b); //output : false
}
}
This is a code sample for using all Relational operators in Java.
Logical operators:
Logical operators are used to compare two operands based on multiple factors. They perform operations on operands and give us results in either true or false values. There are a total of three types of logical operators.
&& (AND) | AND operator will return true if both the operands are true otherwise it will return false. |
|| (OR) | OR operator will return true if either one of the operand is true otherwise it will return false. |
! (NOT) | NOT operator will negates the result of the expression. |
public class DevExpertsHub {
public static void main(String[] args) {
boolean a = true;
boolean b = false;
System.out.println(a&&b); //output : false
System.out.println(a||b); //output : true
System.out.println(!a); //output : false
}
}
Assignment operators:
Assignment operators are used to assign values to the variables. There are many assignment operators
Operator | Sample | Same as |
= | x = 10 | x = 10 |
+= | x += 10 | x = x + 10 |
-= | x -= 10 | x = x – 10 |
*= | x *= 10 | x = x * 10 |
/= | x /= 10 | x = x / 10 |
%= | x %= 10 | x = x % 10 |
&= | x &= 10 | x = x & 10 |
|= | x |= 10 | x = x | 10 |
^= | x ^= 10 | x = x ^ 10 |
>>= | x >>= 10 | x = x >> 10 |
<<= | x <<= 10 | x = x << 10 |
Example 1: x += 10
public class DevExpertsHub {
public static void main(String[] args) {
int x = 10;
x += 10;
// x += x => x = x + 10 (x = 10 + 10)
System.out.println("x value after (x += x) = " + x); // OUTPUT : x value after (x += x) = 20
}
}
Example 2: x -= 10
public class DevExpertsHub {
public static void main(String[] args) {
int x = 10;
x -= 10;
// x += x => x = x - 10 (x = 10 - 10)
System.out.println("x value after (x -= x) = " + x); // OUTPUT : x value after (x -= x) = 10
}
}
Example 3: x *= 10
public class DevExpertsHub {
public static void main(String[] args) {
int x = 10;
x *= 10;
// x *= x => x = x * 10 (x = 10 * 10)
System.out.println("x value after (x *= x) = " + x); // OUTPUT : x value after (x *= x) = 100
}
}
Example 4: x /= 10
public class DevExpertsHub {
public static void main(String[] args) {
int x = 10;
x /= 10;
// x /= x => x = x / 10 (x = 10 / 10)
System.out.println("x value after (x /= x) = " + x); // OUTPUT : x value after (x /= x) = 1
}
}
Example 5: x /= 10
public class DevExpertsHub {
public static void main(String[] args) {
int x = 10;
x %= 10;
// x %= x => x = x % 10 (x = 10 / 10)
System.out.println("x value after (x %= x) = " + x); // OUTPUT : x value after (x %= x) = 0
}
}
Unary operators:
Unary operators in Java are used to perform operations on a single operand. There are a total of 6 types of unary operators in Java.
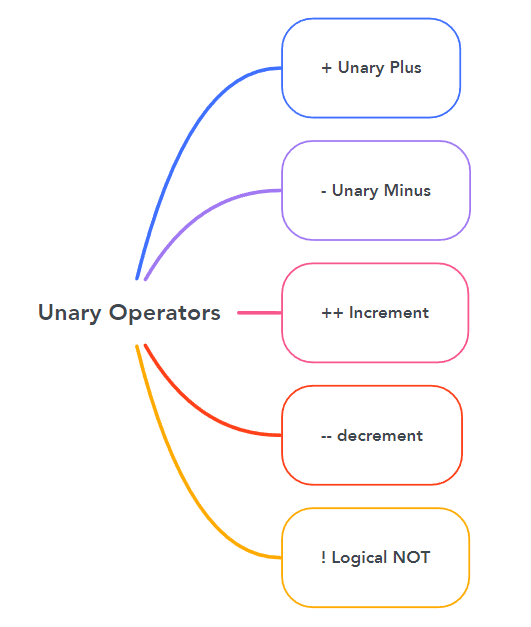
+ (Unary plus) | Indicates a positive number. |
– (Unary minus) | Negates the value. |
++ (Increment) | Increases the value by 1 |
— (decrement) | Decreases the value by 1 |
! (Logical NOT) | Negates the boolean value |
~ (Bitwise NOT) | Inverts the bits of the binary value. It is only applied to integer type values |
Increment and decrement can be used in two forms. They are postfix and prefix.
Postfix: In postfix, the value is returned first and then the value is incremented or decremented. This means the updated value will not be used until the next operation.
Prefix: In prefix, the value is incremented or decremented and then it will return the value. This means the value will be updated immediately in the current operation.
public class DevExpertsHub {
public static void main(String[] args) {
int a = 10;
System.out.println(a++); // OUTPUT : 10 Postfix increment
int b = 10;
System.out.println(++b); // OUTPUT : 11 Prefix increment
int c = 10;
System.out.println(c--); // OUTPUT : 10 Postfix decrement
int d = 10;
System.out.println(--d); // OUTPUT : 9 Prefix decrement
}
}
What is a ternary operator?
The ternary operator in Java is also known as the conditional operator. It means first the condition will be evaluated based on the condition it will return one of the two values.
Syntax : condition ? value_if_true : value_if_false
public class DevExpertsHub {
public static void main(String[] args) {
int age = 23;
String result = (age > 18) ? "You are major" : "You are minor"; // OUTPUT : You are major (because age > 18 so first value is printed)
System.out.println(result);
}
}
What are Bitwise operators?
Bitwise operators are used to perform operations on binary values of integer and long data types. There are a total of 5 types of bitwise operators in Java
- Bitwise AND (&)
- Bitwise OR (|)
- Bitwise XOR (^)
- Bitwise COMPLEMENT (~)
- Shift operators (<<, >>, >>>)
Bitwise AND (&) | Bitwise AND operator will return 1 in each bit position if bits of both operands are 1. |
Bitwise OR (|) | Bitwise OR operator will return 1 in each bit position if either one of their operands is 1. |
Bitwise XOR (^) | Bitwise XOR operator will return 1 if the corresponding bits of two operands are opposite. |
Bitwise COMPLEMENT (~) | Bitwise Complement will flip the bits from 1s to 0s and 0s to 1s. |
Shift operators (<<, >>, >>>) | Shift operators will move the bits left or right by internally multiplying or dividing by powers of 2. |
public class DevExpertsHub {
public static void main(String[] args) {
int a = 12; // 1100 in binary
int b = 7; // 0111 in binary
System.out.println(a&b); // OUTPUT : 4 (0100 in binary)
System.out.println(a|b); // OUTPUT : 15 (1111 in binary)
System.out.println(a^b); // OUTPUT : 11 (1011 in binary)
System.out.println(~a); // OUTPUT : -13 (0011 in binary)
System.out.println(a<<2); // OUTPUT : 48 (110000 in binary)
System.out.println(a>>2); // OUTPUT : 3 (0011 in binary)
System.out.println(a>>>2); // OUTPUT : 3 (0011 in binary)
}
}
Precedence table:
Precedence | Operator | Description |
1 | ( ) | Parentheses |
2 | ++, — | Postfix increment/decrement |
3 | ++, — | Prefix increment/decrement |
4 | +, – | Unary plus/minus |
5 | !, ~ | Logical NOT, bitwise complement |
6 | *, /, % | Multiplication, division, modulus |
7 | +, – | Addition, subtraction |
8 | <<, >>, >>> | Bitwise left shift, bitwise right shift, unsigned bitwise right shift |
9 | <, <=, >, >= | Relational operators |
10 | ==, != | Equality operators |
11 | & | Bitwise AND |
12 | ^ | Bitwise XOR |
13 | | | Bitwise OR |
14 | && | Conditional AND |
15 | || | Conditional OR |
16 | ?: | Ternary operator |
17 | =, +=, -=, *=, /=, %=, &=, ^=, |=, <<=, >>=, >>>= | Assignment operators |