Before directly jumping into data types and variables in Java let’s quickly look into syntax and why we need syntax.
What is syntax and why do we need syntax?
Syntax is a set of rules to follow when writing a program. Different programming languages will have their own syntax and we need to follow their rules for writing the programs. We need syntax because it is the foundation of the programming language it defines how you write and execute the program.
For example, we will look into the syntax of different programming languages for a better understanding. We will try to print “Hello World!” in different programming languages.
Java Code:
public class Main {
public static void main(String[] args) {
System.out.println("Hello World!");
}
}
Python Code:
print("Hello World!")
C++ Code:
#include <iostream>
using namespace std;
int main()
{
cout << "Hello World";
return 0;
}
See every programming language has its own syntax. Now let’s dive into data types.
What are Data Types?
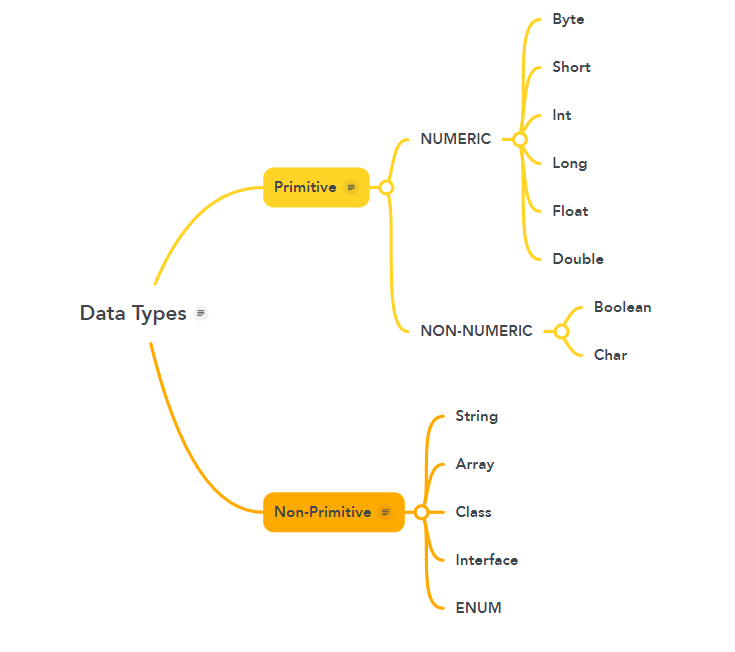
Data types are classified into two types primitive data types and non-primitive data types, Futuremore primitive data types are classified into two types numeric and non-numeric. Let us understand one by one.
Primitive:
There are a total of 8 primitive data types in Java, They are single-value data types and they don’t have any special methods.
- Byte: Byte is a data type that will store whole numbers from the range -128 to 127, and the data type size is 1 byte, which means 1 byte of size will be stored in the memory.
byte a = 2; // Stores Integer values from range -128 to 127
- Short: Short is a data type that will store whole numbers from the range -32,768 to 32,767, and the data type size is 2 bytes, which means 2 bytes of size will be stored in the memory.
short a = 20; // Stores Integer values from range -32,768 to 32,767
- Int: Int is a data type that will store whole numbers from the range -2,147,483,648 to 2,147,483,647, and the data type size is 4 bytes, which means 4 bytes of size will be stored in the memory.
int a = 10; // Stores Integer values from range -2,147,483,648 to 2,147,483,647
- Long: Long is a data type that will store whole numbers from the range -9,223,372,036,854,775,808 to 9,223,372,036,854,775,807, and the data type size is 8 bytes, which means 8 bytes of size will be stored in the memory.
long a = 100; // Stores Integer values from range -9,223,372,036,854,775,808 to 9,223,372,036,854,775,807
- Float: Float is a data type that will store decimal numbers up to 7 decimal points, and the data type size is 4 bytes, which means 4 bytes of size will be stored in the memory.
float a = 3.14f; // Stores decimal points
- Double: Double is a data type that will store decimal numbers up to 15 decimal points, and the data type size is 8 bytes, which means 8 bytes of size will be stored in the memory.
double a = 7.47; // Stores decimal points
- Boolean: Boolean will store only two values, either true or false, and the data type size is 1 byte, which means 1 byte of size will be stored in memory.
boolean isPresent = true; // Stores only true or false values
- Char: Char will store only a single character or letter, and the data type size is 2 bytes, which means 2 bytes of size will be stored in memory.
char ch = 'A'; // Stores single letter
Non-Primitive:
- String: A string is a sequence of characters. A string is an object in Java and we have many methods that can use for multiple purposes. A string can have a null value. Only string is provided by Java remaining all non-primitive data types are user-defined data types.
String name = "DevExpertsHub"; // Stores sequence of characters
- Array: Arrays will store multiple values in continuous memory locations of similar data type. we must specify the length of the array will creating an array.
ArrayList<Integer> array = new ArrayList<Integer>(); // Stores list of values in continuous memory locations
- Classes: A class is a blueprint or user-defined prototype. we can create objects based on class. A class will define a set of attributes and properties that all objects of that particular class will have.
public class Main { // Main is the class name
public static void main(String[] args) {
System.out.println("Welcome to DevExpertsHub");
}
}
- Interface: Interface is a blueprint of a class. Generally, we define an interface for achieving abstraction and all methods inside the interface will have only a method definition but nobody. By using an interface we can achieve multiple inheritance.
public interface Animal{ // Animal is interface name
void sleeping(); // only method definition but nobody
}
- ENUM: ENUM is somewhat similar to a class but we cannot create an object of it and we cannot extend ENUM. ENUMs are constants that are public, static, and final means they cannot change or be overridden.
enum days{
MONDAY,
TUESDAY,
WEDNESDAY,
THURSDAY,
FRIDAY,
SATURDAY,
SUNDAY
}
What are Variables in Java?
Variables are containers that store the values in memory location during the run time of the program. A variable is used for the memory location name for that value. A variable is defined with data type followed by variable name.
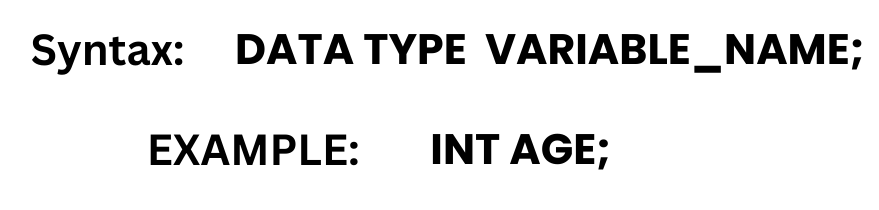
There are a total of three types of variables in Java.
- Local variable
- Instance variable
- static variable
Local variables:
Local variables are declared inside the method and accessible only inside the method. we cannot use local variables outside the method. A local variable cannot be defined with a static keyword.
Instance variables:
Instance variables are declared inside the class and outside the method these kinds of variables are known as instance variables. Instance variables are used with that particular instance and other instances cannot use these variables.
Static variables:
Static variables are defined using the static keyword. we can create one static variable and use it for all instances of the particular class. Static variables are created during the run time of the program. These static variables are defined inside the class and outside the method.
public class Main {
public static boolean isPresent = true; // Static variable
String name = "DevExpertsHub"; // Instance variable
public static void main(String[] args) {
System.out.println(Main.isPresent); // Printing Static variable
}
public void greeting(){
int age = 23; // Local variable
System.out.println("My age is " + age); // Printing local variable
System.out.println("Welcome to "+name); // Printing Instance variable
}
}
What are Identifiers?
An identifier in Java is a name given to a variable, method, class, interface, package, or any other user-defined item. Identifiers are used to uniquely identify our items in Java code. They improve the readability and understandability of our code.
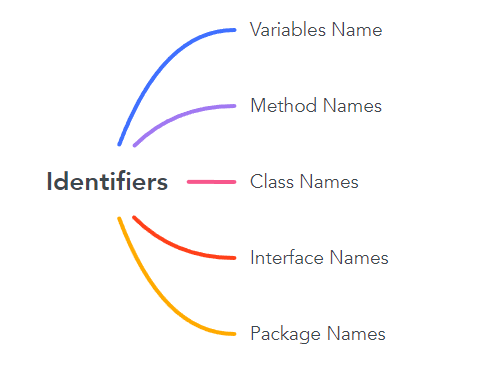
Rules for defining an identifier:
- Every identifier has to start with (A to Z or a to z), $(Dollar), or _ (underscore).
- We can’t use any other special characters for creating an identifier. Only _ (underscore) and $ (Dollar) characters can be used.
- Java keywords cannot be defined as identifiers. For example, we cannot use (int, float, long, etc).
- Identifiers are case-sensitive. For example, age, Age, and AGE are all different.
Assignment:
- Write a Java program to create all primitive data types and print those values.
- Write a Java program to create a variable using _ (underscore) as the starting of the variable name and print it.